Lag en enkel videospiller med html, CSS og Javascript
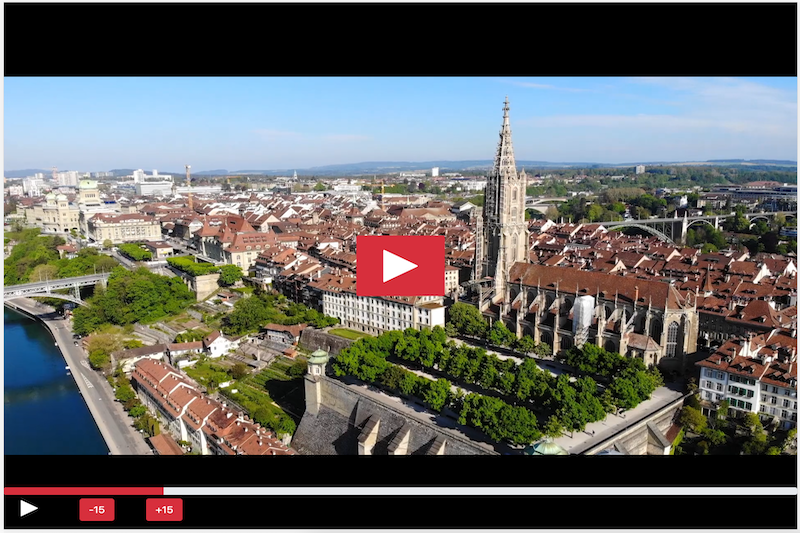
Lag en enkel videospiller med html, CSS og Javascript
av Ole Petter den 10. mars 2021
Sist oppdatert: 26 april, 2021 kl 13:49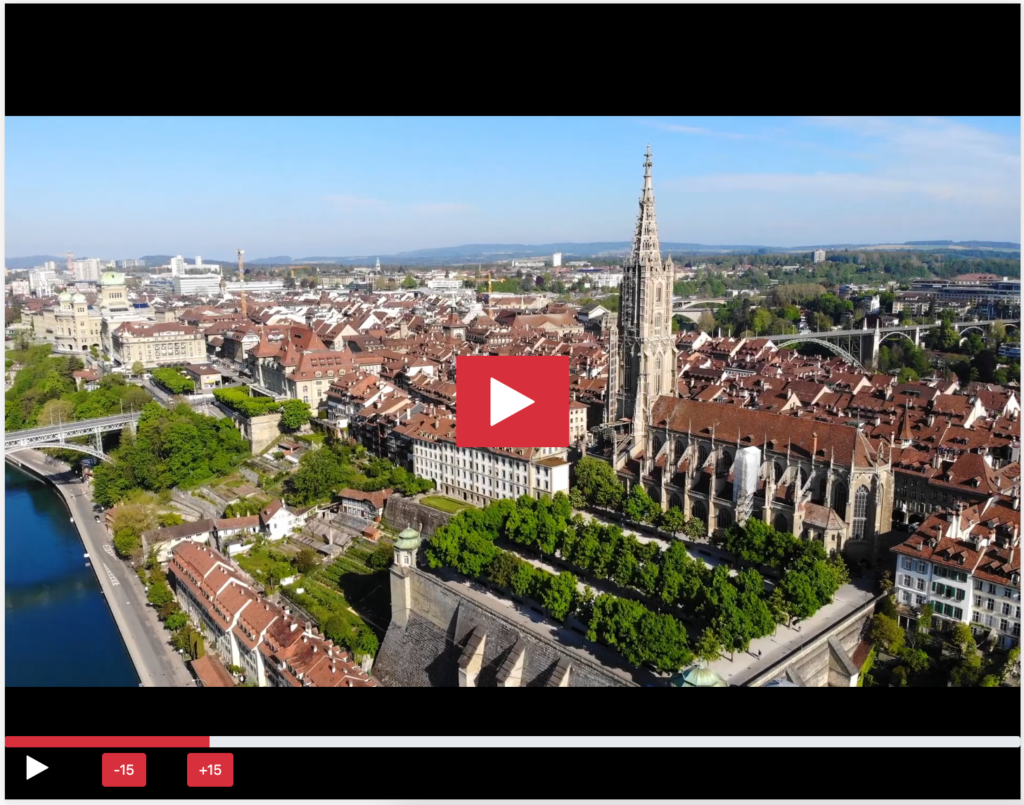
Contents
Live versjon
https://progitek.no/ressurser/nettsider/videoplayer/
index.html
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<link rel="stylesheet" href="style.css" />
<link
rel="stylesheet"
href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"
/>
<link
rel="stylesheet"
href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"
/>
</head>
<body>
<div class="videoContainer">
<video id="video">
<source src="video.mp4" type="video/mp4" />
</video>
<div class="playPauseOverlay" id="playPauseOverlay">
<button
class="btn btn-danger px-4 py-3"
id="playPauseOverlayButton"
>
<i class="fa fa-play fa-5x text-white"></i>
</button>
</div>
<div class="videoControls mb-3">
<div class="row">
<div class="col-12">
<div class="progress" id="progress">
<div
class="progress-bar bg-danger"
id="progressBar"
role="progress"
style="width: 0%"
aria-valuenow="25"
aria-valuemin="0"
aria-valuemax="100"
></div>
</div>
</div>
<div class="col-1 text-center">
<button id="playButton" class="btn btn-lg">
<i class="fa fa-play text-white fa-2x"></i>
</button>
</div>
<div class="col-1 text-center">
<button
class="btn btn-lg btn-danger mt-2"
id="skipBackwardButton"
>
-15<i class="fa fa-redo text-white"></i>
</button>
</div>
<div class="col-1 text-center">
<button
class="btn btn-lg btn-danger mt-2"
id="skipForwardButton"
>
+15<i class="fa fa-redo text-white"></i>
</button>
</div>
</div>
</div>
</div>
<script src="app.js"></script>
</body>
</html>
app.js
const playButton = document.getElementById("playButton")
const videoRef = document.getElementById("video")
const progressBar = document.getElementById("progressBar")
const progressContainer = document.getElementById("progress")
const skipForward = document.getElementById("skipForwardButton")
const skipBackward = document.getElementById("skipBackwardButton")
const playPause = document.getElementById("playPauseOverlayButton")
const playPauseOverlay = document.getElementById("playPauseOverlay")
videoRef.addEventListener("click", () => {
handlePlay()
})
playPauseOverlay.addEventListener("click", () => {
handlePlay()
})
progressContainer.addEventListener("click", (e) => {
console.log(e)
})
skipBackward.addEventListener("click", () => {
videoRef.currentTime = videoRef.currentTime - 15
})
skipForward.addEventListener("click", () => {
videoRef.currentTime = videoRef.currentTime + 15
})
setInterval(() => {
//console.log(videoRef.currentTime, videoRef.duration)
progressBar.style.width = ((videoRef.currentTime / videoRef.duration) * 100) + "%"
if (videoRef.ended || videoRef.paused) {
playButton.innerHTML = "<i class='fa fa-play text-white fa-2x'></i>"
}
}, 100)
function handlePlay() {
if (video.paused) {
video.play()
playButton.innerHTML = "<i class='fa fa-pause text-white fa-2x'></i>"
playPauseOverlay.innerHTML = `
<button
class="btn btn-danger px-4 py-3"
id="playPauseOverlayButton"
>
<i class="fa fa-pause fa-5x text-white"></i>
</button>`
setTimeout(() => {
playPauseOverlay.innerHTML = ""
}, 1500)
} else {
video.pause()
playButton.innerHTML = "<i class='fa fa-play text-white fa-2x'></i>"
playPauseOverlay.innerHTML = `
<button
class="btn btn-danger px-4 py-3"
id="playPauseOverlayButton"
>
<i class="fa fa-play fa-5x text-white"></i>
</button>`
/* setTimeout(() => {
playPauseOverlay.innerHTML = ""
}, 1500) */
}
}
style.css
video {
max-width: 100%;
height: 100%;
}
.videoContainer {
position: fixed;
top: 0px;
left: 0px;
height: 100%;
width: 100%;
background-color: black;
}
.videoControls {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
}
.progress {
margin-top: 20px;
width: 100%;
}
.playButton {
/* margin-bottom: 100px; */
}
.playPauseOverlay {
position: absolute;
left: 50%;
top: 50%;
z-index: 10;
}
Legg igjen en kommentar
Søk på siden
Siste innlegg
Siste kommentarer
Arkiv
Kategorier